I have been using Chakra UI in a personal project. So far, I'm liking the library a lot since it has many similarities with Tailwind CSS. However, I found out that there is no property for making the Drawer component always open. After some searching, I discovered a solution.
To introduce the issue, the drawer is wrapped by a container div
, which occupies the whole screen, blocking all click events to the underlying elements.
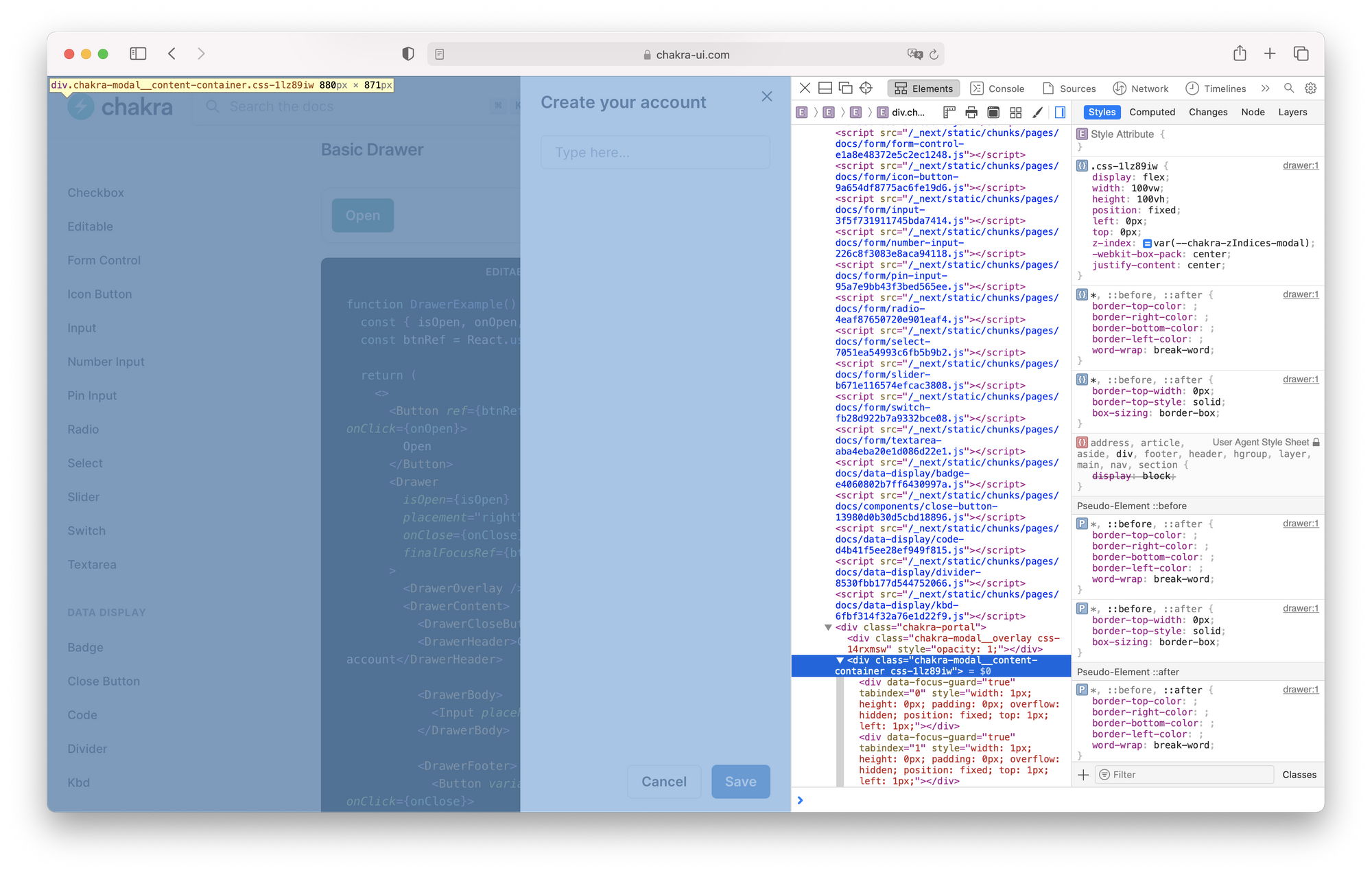
GitHub user jasonwarta came up with a workaround that works by extending Chakra's default theme. Note that I have done some minor changes to their code.
First, we extend the Drawer component using the function extendTheme
, creating a variant with the desired behavior - I called it permanent. This solution basically lets the pointer events go through the drawer's container, using the CSS property pointer-events
.
// theme.ts
import { extendTheme } from '@chakra-ui/react';
const theme = extendTheme({
components: {
Drawer: {
variants: {
permanent: {
dialog: {
pointerEvents: 'auto',
},
dialogContainer: {
pointerEvents: 'none',
},
},
},
},
},
});
export default theme;
Then, we configure the ChakraProvider
to use the created theme.
import { ChakraProvider } from '@chakra-ui/react';
<ChakraProvider theme={theme}>
// ...
</ChakraProvider>
At last, just pass the new variant as a property to your Drawer component.
const MyComponent = ({ children }) => {
return (
<Drawer variant="permanent">
<DrawerContent>
<DrawerHeader borderBottomWidth="1px">Drawer Header</DrawerHeader>
<DrawerBody>Drawer Body</DrawerBody>
</DrawerContent>
</Drawer>
);
};
In a similar way, I created another solution, although I haven't tested it thoroughly yet. Instead of working with pointer-events
, I just set the width of the container to zero.
// theme.ts
import { extendTheme } from '@chakra-ui/react';
const theme = extendTheme({
components: {
Drawer: {
variants: {
permanent: {
dialogContainer: {
width: 0,
},
},
},
},
},
});
export default theme;
If you find this post helpful, do not forget to give a thumbs up to the original solution!