As a software developer, I create a lot of new projects that I end up dropping, and sometimes I feel that configuring a new project is a hassle and makes me lose interest. That happened to me with React as there are several libraries I install and configure whenever I create a new app, namely TypeScript, CRACO, ESLint, Prettier, Sass and Tailwind CSS. To solve this issue, I created a list of steps to set up a new React project with the help of create-react-app
, which led me to a template repository I can reuse to kickstart a workspace.
Creating a new React app
First steps first, let's create a new React app through create-react-app
. Notice that I set the template flag to the TypeScript template; you can use any other template, or omit the flag to install the default JavaScript template.
# npm <= 6
npx create-react-app react-template --template typescript
# npm >= 7
npm exec -- create-react-app react-template --template typescript
Install CRACO
CRACO, or Create React App Configuration Override, is "an easy and comprehensible configuration layer for create-react-app". We will use this utility to configure ESLint and PostCSS, the latter to be able to use Tailwind CSS. Just install it using npm
.
npm install @craco/craco
Following CRACO's instructions, "update the existing calls to react-scripts
in the scripts
section of your package.json
file to use the craco
CLI":
/* package.json */
"scripts": {
- "start": "react-scripts start",
+ "start": "craco start",
- "build": "react-scripts build",
+ "build": "craco build"
- "test": "react-scripts test",
+ "test": "craco test"
}
Let's create a CRACO config file, craco.config.js
. It will be empty for now, we will soon add to it.
// craco.config.js
module.exports = {};
Adding ESLint rules
React projects created through create-react-app
come with an ESLint configuration by default. However, I think it is not enough as I am a fan of opinionated environments (that's one of the reasons I like Angular and NestJS).
One of the most famous set of rules for React development is the Airbnb configuration which enforces Airbnb's JavaScript style guide. There is a community package that brings TypeScript support to this configuration: eslint-config-airbnb-typescript
. Start by installing it as a dev dependency.
npm install eslint-config-airbnb-typescript --save-dev
Then, we have to install some plugins for it to work. There is a limitation with ESLint, see RFC and progress for more information.
npm install eslint-plugin-import@^2.22.0 eslint-plugin-jsx-a11y@^6.3.1 eslint-plugin-react@^7.20.3 eslint-plugin-react-hooks@^4.0.8 @typescript-eslint/eslint-plugin@^4.4.1 --save-dev
If you are having issues installing it using npm >= 7
because of npm
not being able to resolve the dependency tree, just add the flag --legacy-peer-deps
to the command - it will ensure the peer dependencies are not installed automatically.
Now, create an ESLint configuration file, .eslintrc.js
, and add the following content to it:
// .eslintrc.js
module.exports = {
env: {
browser: true,
es2021: true,
},
extends: [
'plugin:react/recommended',
'airbnb-typescript',
],
parser: '@typescript-eslint/parser',
parserOptions: {
ecmaFeatures: {
jsx: true,
},
ecmaVersion: 12,
project: './tsconfig.json',
sourceType: 'module',
},
plugins: ['react', '@typescript-eslint']
};
Let's not forget to point the CRACO configuration file to our ESLint configuration file.
// craco.config.js
const { ESLINT_MODES } = require('@craco/craco');
module.exports = {
eslint: {
mode: ESLINT_MODES.file,
},
};
Turn your code pretty with Prettier
I can't state enough how much I love Prettier and its Visual Studio Code extension. I have the "Format on Save" option on all the time, and with the help of Prettier I always have my code perfectly organized.
Install the following dependencies in your app.
npm i prettier eslint-config-prettier eslint-plugin-prettier --save-dev
Extend your ESLint configuration with the installed configuration and plugin. Add also a custom rule to show errors if Prettier rules are not followed.
// .eslintrc.js
module.exports = {
env: {
browser: true,
es2021: true,
},
extends: [
'plugin:react/recommended',
'airbnb-typescript',
+ 'prettier',
],
parser: '@typescript-eslint/parser',
parserOptions: {
ecmaFeatures: {
jsx: true,
},
ecmaVersion: 12,
project: './tsconfig.json',
sourceType: 'module',
},
- plugins: ['react', '@typescript-eslint'],
+ plugins: ['react', '@typescript-eslint', 'prettier'],
+ rules: {
+ 'prettier/prettier': 'error',
+ },
};
If you wish, create also a .prettierrc
file to configure Prettier in VS Code. This is my default configuration:
{
"singleQuote": true,
"tabWidth": 2,
"trailingComma": "all"
}
It's possible that you find some Prettier errors after this step - just fix them accordingly!
Style your app with Tailwind CSS
Tailwind CSS is a marvel for styling your apps. While most CSS frameworks are component based (e.g. Bootstrap, Bulma), Tailwind CSS is an utility-first framework, with classes for practically everything - text colors, layouts, animations, etc.
Begin by installing the required packages. As of this writing, create-react-app
still uses PostCSS 7, but Tailwind CSS 2 requires PostCSS 8. As such, we have to install the compatibility build of Tailwind CSS.
npm i tailwindcss@npm:@tailwindcss/postcss7-compat @tailwindcss/postcss7-compat autoprefixer@^9
After installing, override the default React PostCSS config with the help of CRACO:
// craco.config.js
const { ESLINT_MODES } = require('@craco/craco');
+ const autoprefixer = require('autoprefixer');
+ const tailwind = require('tailwindcss');
module.exports = {
eslint: {
mode: ESLINT_MODES.file,
},
+ style: {
+ postcss: {
+ plugins: [autoprefixer, tailwind],
+ },
+ },
};
Now, we need to configure Tailwind CSS to remove unused styles in production so it does not create an oversized bundle. Create a tailwind.config.js
file in the root of your project and add the following code:
// tailwind.config.js
module.exports = {
purge: ['./src/**/*.{js,jsx,ts,tsx}', './public/index.html'],
};
Finally, include Tailwind in your main CSS file.
// src/index.css
@tailwind base;
@tailwind components;
@tailwind utilities;
Check if it is working by changing any class name in your app. I added text-red-400
as the class name to the default paragraph in App.tsx
.
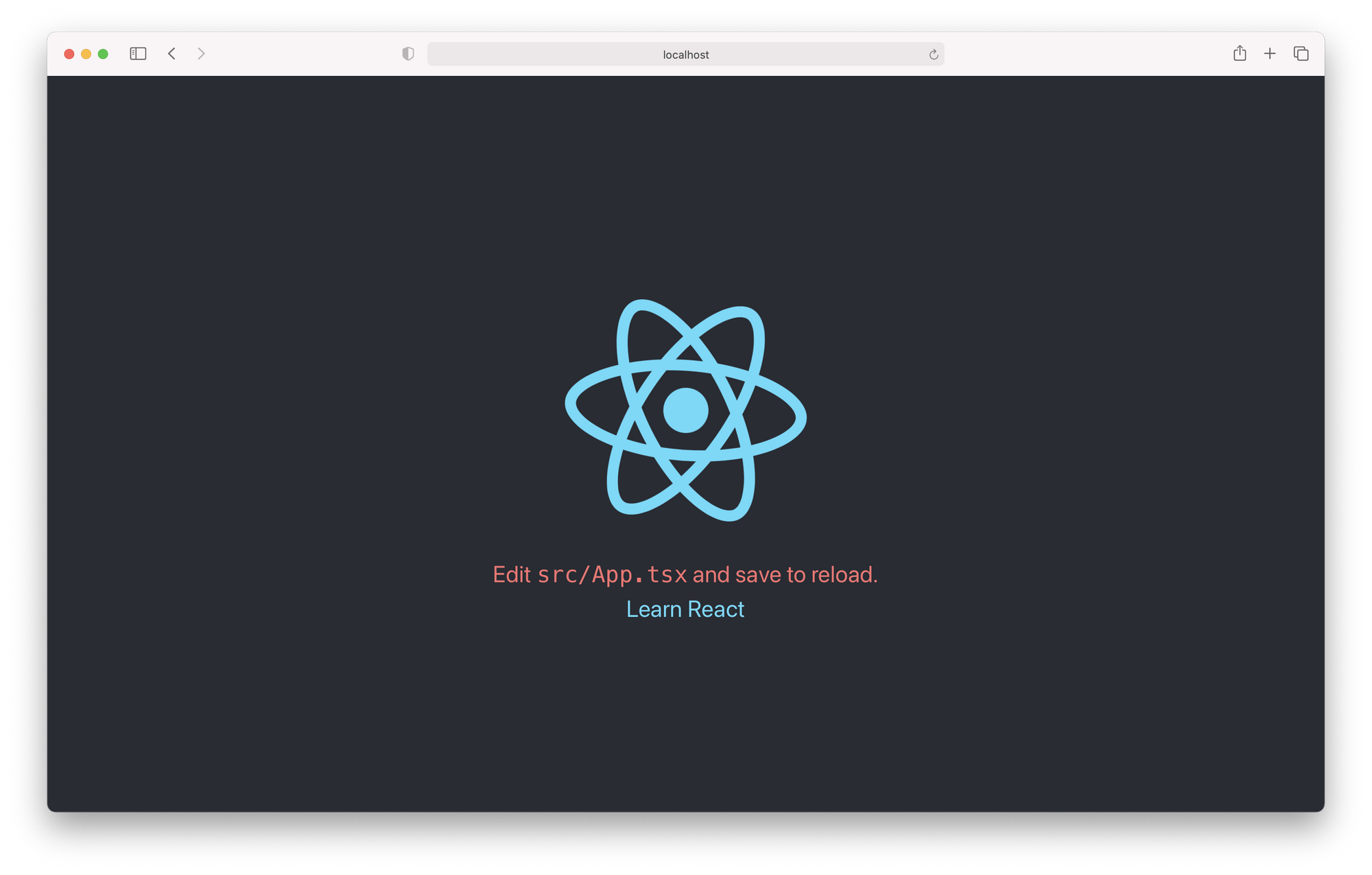
Power up your CSS with Sass
There's a habit I kept from all the time I spent working with Angular, which is using SCSS for styling my apps. It is a mix of the powerful features from Sass and the known CSS syntax, which makes it easy to learn and use.
Install the sass
package in your project, and you can start changing your CSS files to SCSS or Sass files! Also, do not forget to change the imports in your .tsx/.jsx
files.
npm i sass
Final thoughts
It can be tiresome to configure a new React project, more so if it is the first time since some research is required for some problems that may occur. With the template I created through these steps, I want to minimize the hassle of creating new React apps.
If you have any suggestions or questions, please feel free to open an issue in the linked repository.